MongoDB is one the most popular NOSQL databases. It is a document oriented database. That means you will be inserting/deleting/updating/querying documents instead of rows. Documents allows you to represent hierarchical relationships within a single document and this naturally fits the way how we use objects in an object oriented programming language. Also you do not have to use a strict schema for your documents, you can evolve structure of your documents when you need. For example this is a document:
{'name' : 'Deniz Oguz'}
Documents are stored in collections which are analogical to tables in relational databases except each document could be different even within a collection. Using different collections for each category of documents makes it easier to develop programs. MongoDB also supports subcollections as a syntactical sugar. You can create namespaces using ‘.’ notation to group your schemas. For example: school.courses, school.students are different collections but just they share a common prefix as their collection name. Each installation of MongoDB can contain multiple databases each of which consist of collections.
Later we will dive details of documents and collections but before we proceed I would like to get MongoDB running and save and query documents from it.
Prepare Your Environment
We will prepare our development environment by downloading and installing neccesssary tools.
- MongoDB server
- Database frontend
- Driver for your programming language
Server
Download MongoDB Server. Instructions for installing MongoDB on all three platforms are available at http://docs.mongodb.org/manual/installation so we do not need to repeat it here.
Database Frontend
MongoDB has its own commandline interface but since you are reading this introduction you should use one of the following GUI tools.
Mac OS X
MongoHub: It is free and could be downloaded from http://mongohub.todayclose.com/. It even has import/export capability from MySQL.
Windows
MongoVUE: It has a free single user version and can be downloaded from http://www.mongovue.com/downloads/.

Linux & Platform Independt Frontend
UMongo is a java based MongoDB frontend. You can download it from http://www.girbal.net/umongo/

Eclipse Plugin
You can use MonjaDB plugin. Download it from here.

Drivers
You can download MongoDB drivers from http://docs.mongodb.org/ecosystem/drivers/. Since we will use Node.js and JavaScript for this tutorial I will give instructions for Mongoose. Mongoose is actually not a driver, it is object mapping library build on top of MongoDB Node.js driver. Of course you should install Node.js first. Grap the Node.js installar of your platform from http://nodejs.org/download/ and install it. After Node.js is installed install Mongoose using npm.
npm install mongoose
This command will install mongoose and its dependencies into node_modules folder in the directory you invoke the command. Parent directory of node_modules directory will be our working folder.
Development with MongoDB
Most basic example of using a database is saving something to it and reading it back. So will save a document to MongoDB and query this document back from it. Of course we have to start MongoDB server before.
Starting MongoDB Server
mongod assumes default data folder as /data/db so make sure it is exist or give a path of existing directory with –dbpath. It uses default port 27017 for connections, it will also start an admin web console on port 28017. Since this is an introductory tutorial there is nothing interesting in web console for us. It given extensive statistics on MongoDB server.
starscream-5:bin starscream$ ./mongod --dbpath ~/mongodb-osx-x86_64-2.4.4/data/
Mon Jun 24 00:13:56.309 [initandlisten] MongoDB starting : pid=532 port=27017 dbpath=/Users/starscream/mongodb-osx-x86_64-2.4.4/data/ 64-bit host=starscream-5.local
Mon Jun 24 00:13:56.310 [initandlisten]
Mon Jun 24 00:13:56.310 [initandlisten] ** WARNING: soft rlimits too low. Number of files is 256, should be at least 1000
Mon Jun 24 00:13:56.310 [initandlisten] db version v2.4.4
Mon Jun 24 00:13:56.310 [initandlisten] git version: 4ec1fb96702c9d4c57b1e06dd34eb73a16e407d2
Mon Jun 24 00:13:56.310 [initandlisten] build info: Darwin bs-osx-106-x86-64-2.10gen.cc 10.8.0 Darwin Kernel Version 10.8.0: Tue Jun 7 16:32:41 PDT 2011; root:xnu-1504.15.3~1/RELEASE_X86_64 x86_64 BOOST_LIB_VERSION=1_49
Mon Jun 24 00:13:56.310 [initandlisten] allocator: system
Mon Jun 24 00:13:56.310 [initandlisten] options: { dbpath: "/Users/starscream/mongodb-osx-x86_64-2.4.4/data/" }
Mon Jun 24 00:13:56.316 [initandlisten] journal dir=/Users/starscream/mongodb-osx-x86_64-2.4.4/data/journal
Mon Jun 24 00:13:56.316 [initandlisten] recover begin
Mon Jun 24 00:13:56.317 [initandlisten] recover lsn: 54971
Mon Jun 24 00:13:56.317 [initandlisten] recover /Users/starscream/mongodb-osx-x86_64-2.4.4/data/journal/j._0
Mon Jun 24 00:13:56.319 [initandlisten] recover skipping application of section seq:0 < lsn:54971
Mon Jun 24 00:13:56.320 [initandlisten] recover create file /Users/starscream/mongodb-osx-x86_64-2.4.4/data/test.ns 16MB
Mon Jun 24 00:13:56.370 [initandlisten] recover create file /Users/starscream/mongodb-osx-x86_64-2.4.4/data/test.0 64MB
Mon Jun 24 00:13:56.546 [initandlisten] recover cleaning up
Mon Jun 24 00:13:56.546 [initandlisten] removeJournalFiles
Mon Jun 24 00:13:56.548 [initandlisten] recover done
Mon Jun 24 00:13:56.704 [websvr] admin web console waiting for connections on port 28017
Mon Jun 24 00:13:56.704 [initandlisten] waiting for connections on port 27017
Saving Document to MongoDB
Create a file saveToMongoDB.js with your favorite text editor. We will write a most basic thing with MongoDB, save a simple document to it.
1 var mongoose = require'mongoose'; 2 3 //like all nodejs libraries mongoose also works asynchronously with call back functions 4 //result of connect function will be available to our callback function 5 //connect takes URL of the DB and a callback. Name of DB we are connecting is test 6 //DB does not have to be exist, it will be created automatically 7 var connection = mongoose connect'mongodb://localhost/test'function err 8 if err 9 throw err; 10 11 console.log'Connected to MongoDB'; 12 13 //Define a schema for your document 14 var messageSchema = 15 from : String 16 to: String 17 subject : String 18 body : String 19 deliveryTime : type: Datedefault: Date now 20 ; 21 22 //create Model class for your model 23 var MessageModel = mongoose model'MessageModel' messageSchema; 24 25 //create an instance of your actual model/document 26 var message = 27 from : 'Deniz Oğuz' 28 to : 'Ata Oğuz' 29 subject : 'Furby' 30 body : 'Furby yi aldım oğluş.' 31 deliveryTime : 32 ; 33 34 //save your document/model to MongoDB 35 //again we have supply call back function to process result 36 message savefunction err msg 37 if err 38 throw err; 39 40 console.log"Saved:" + msg _id;//every document has '_id' field generated by mongodb 41 ; 42 ; 43
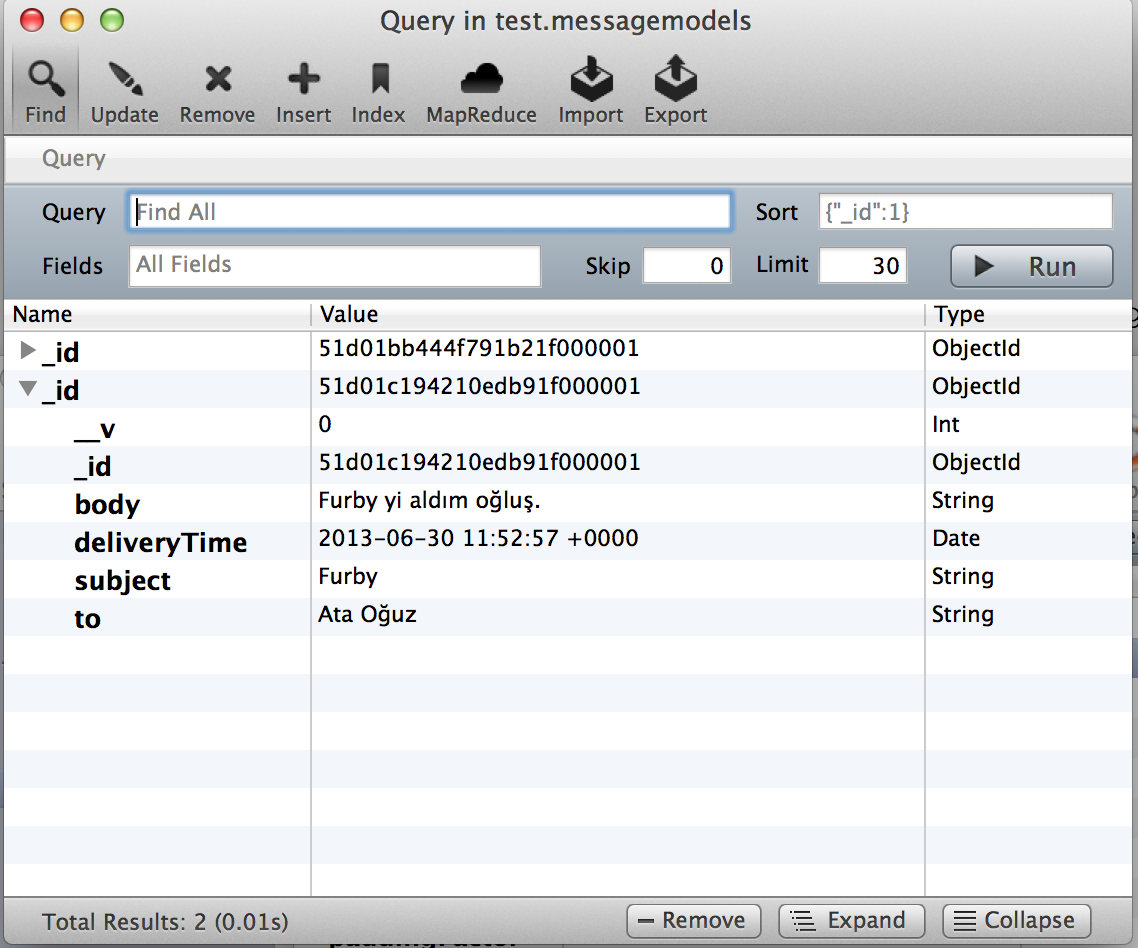
Query Documents from MongoDB
Now create a new file queryMongoDB.js. We will query MongoDB and find all documents matching our query and print subject of each document. It is very similar to above so I am not repeating the common parts, everything up to MessageModel.save will be the same. Replace call to save method with the following code block.
1 console.log'querying...'; 2 MessageModelfind{}function err messages 3 if err 4 throw err; 5 6 for i = 0; i < messageslength; i++ 7 console.log'message:' + messages i subject; 8 ;